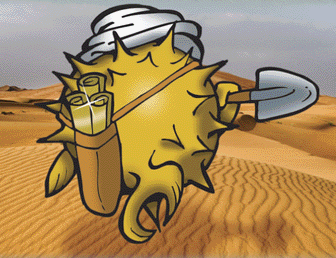
read CAT source file
我学习cat命令的源码及注释。
/* $OpenBSD: cat.c,v 1.20 2009/10/27 23:59:19 deraadt Exp $ */ /* $NetBSD: cat.c,v 1.11 1995/09/07 06:12:54 jtc Exp $ */ /* * Copyright (c) 1989, 1993 * The Regents of the University of California. All rights reserved. * * This code is derived from software contributed to Berkeley by * Kevin Fall. * * Redistribution and use in source and binary forms, with or without * modification, are permitted provided that the following conditions * are met: * 1. Redistributions of source code must retain the above copyright * notice, this list of conditions and the following disclaimer. * 2. Redistributions in binary form must reproduce the above copyright * notice, this list of conditions and the following disclaimer in the * documentation and/or other materials provided with the distribution. * 3. Neither the name of the University nor the names of its contributors * may be used to endorse or promote products derived from this software * without specific prior written permission. * * THIS SOFTWARE IS PROVIDED BY THE REGENTS AND CONTRIBUTORS ``AS IS'' AND * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE * ARE DISCLAIMED. IN NO EVENT SHALL THE REGENTS OR CONTRIBUTORS BE LIABLE * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS * OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) * HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY * OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF * SUCH DAMAGE. */ #include <sys/param.h> #include <sys/stat.h> #include #include #include #include #include #include #include #include #include extern char *__progname; /* 使用extern表示变量来自另外一个源文件 */ int bflag, eflag, nflag, sflag, tflag, vflag; int rval; char *filename; void cook_args(char *argv[]); void cook_buf(FILE *); void raw_args(char *argv[]); void raw_cat(int); int main(int argc, char *argv[]) { int ch; setlocale(LC_ALL, ""); /* setlocale为库中某些特定的例程(routines)设定自然语言的显示格式。 每种这样的显示格式称为一个locale * 用法:setlocale(int category, const char *locale); 其中第一个参数LC_ALL类(category)定义全部通用的显示格式; 其他的还有LC_COLLATE,LC_CTYPE,LC_MONETRAY等。 第二个参数为空“”,表示程序调用时所处的环境(native environment); 其他的还有“C”,“POSIX"。它们是默认的三种locale 如果参数中category和locale是一个没有意义的组合, setlocale()返回一个空指针(NULL) */ while ((ch = getopt(argc, argv, "benstuv")) != -1) /* getopt用于获取cat命令的参数,有效参数为benstuv中的一个或多个 */ switch (ch) { case 'b': /* 不计算空白行,且输出行号 */ bflag = nflag = 1; /* -b implies -n */ break; /* 跳出当前的switch循环 */ case 'e': /* 在行尾输出$字符,且显示非打印字符(=v)*/ eflag = vflag = 1; /* -e implies -v */ break; case 'n': /* 输出行号 */ nflag = 1; break; case 's': /* 合并多个相邻的空白行 */ sflag = 1; break; case 't': /* 将tab字符显示为^I,且显示非打印字符 */ tflag = vflag = 1; /* -t implies -v */ break; case 'u': /* 保证输出不被缓冲 */ setbuf(stdout, NULL); break; case 'v': /* 显示非打印字符 */ vflag = 1; break; default: (void)fprintf(stderr, "usage: %s [-benstuv] [file ...]\n", __progname); exit(1); /* NOTREACHED */ } argv += optind; /* optind 用于多次调用getopt;第一次从getopt返回后, optind含有指向下一个argv参数的索引 * optind初始化为1 * The variables "opterr" and "optind" are both initialized to 1. The optind * variable may be set to another value larger than 0 before a set of calls * to getopt() in order to skip over more or less argv entries. An optind * value of 0 is reserved for compatibility with GNU getopt(). */ if (bflag || eflag || nflag || sflag || tflag || vflag) cook_args(argv); /* 如果有参数就执行cook_args函数*/ else raw_args(argv); /* 如果没有参数就执行raw_args函数*/ if (fclose(stdout)) err(1, "stdout"); exit(rval); /* NOTREACHED */ } void cook_args(char **argv) { FILE *fp; fp = stdin; filename = "stdin"; /* starting a do...while loop ... */ do { if (*argv) { if (!strcmp(*argv, "-")) / * strcmp,如果两个字符相同则返回0 */ fp = stdin; else if ((fp = fopen(*argv, "r")) == NULL) { warn("%s", *argv); /* warn 用于在stderr上显示格式化的错误信息 */ rval = 1; ++argv; continue; /* 结束本次循环,即跳过循环体下面尚未执行的语句,接着进行下一次是否执行循环的判断, * (就是执行到continue时,立即结束本次循环,重新去判断循环条件是否为真) */ } filename = *argv++; } cook_buf(fp); if (fp == stdin) clearerr(fp); /* clearerr的作用是清楚fp的EOF和错误标志*/ else (void)fclose(fp); } while (*argv); } /* end of a do...while loop ... */ void cook_buf(FILE *fp) { int ch, gobble, line, prev; line = gobble = 0; for (prev = '\n'; (ch = getc(fp)) != EOF; prev = ch) { /* prev = '\n'; while (ch = getc(fp) != EOF) { ....;prev = ch;} */ if (prev == '\n') { /* 第一个IF */ if (sflag) { /* 第二个IF */ /* 如果设置sflag=1,表示多个相邻的空白行要合并成一个*/ if (ch == '\n') { if (gobble) continue; gobble = 1; } else /* else和离它最近的一个没有else的IF关键词结合,这里应该是if (ch == '\n'), 如果有大括号进行分段则以大括号为准 */ gobble = 0; } /* 第二个IF 结束*/ if (nflag && (!bflag || ch != '\n')) { /* nflag 控制是否显示行号, bflag也是要求显示行号,但不计算空白行 */ (void)fprintf(stdout, "%6d\t", ++line); /* fprintf中“%6d\t"这种格式是要将打印的变量至少占6个字符的宽度, 不够6个字符的情况下前面补上空格 */ if (ferror(stdout)) /* ferror函数测试文件流的错误指示标志(error indicator), 如果标志已经设置则返回非0值 */ break; } } /* 第一个IF 结束*/ if (ch == '\n') { if (eflag && putchar('$') == EOF) break; } else if (ch == '\t') { if (tflag) { if (putchar('^') == EOF || putchar('I') == EOF) break; continue; } } else if (vflag) { if (!isascii(ch)) { if (putchar('M') == EOF || putchar('-') == EOF) break; ch = toascii(ch); } if (iscntrl(ch)) { if (putchar('^') == EOF || putchar(ch == '\177' ? '?' : ch | 0100) == EOF) break; continue; } } if (putchar(ch) == EOF) break; } /* FOR循环结束 */ if (ferror(fp)) { warn("%s", filename); rval = 1; clearerr(fp); } if (ferror(stdout)) err(1, "stdout"); } void raw_args(char **argv) { int fd; fd = fileno(stdin); filename = "stdin"; do { if (*argv) { if (!strcmp(*argv, "-")) fd = fileno(stdin); else if ((fd = open(*argv, O_RDONLY, 0)) < 0) { warn("%s", *argv); rval = 1; ++argv; continue; } filename = *argv++; } raw_cat(fd); if (fd != fileno(stdin)) (void)close(fd); } while (*argv); } void raw_cat(int rfd) { int wfd; ssize_t nr, nw, off; static size_t bsize; static char *buf = NULL; struct stat sbuf; wfd = fileno(stdout); if (buf == NULL) { if (fstat(wfd, &sbuf)) err(1, "stdout"); bsize = MAX(sbuf.st_blksize, BUFSIZ); if ((buf = malloc(bsize)) == NULL) err(1, "malloc"); } while ((nr = read(rfd, buf, bsize)) != -1 && nr != 0) for (off = 0; nr; nr -= nw, off += nw) if ((nw = write(wfd, buf + off, (size_t)nr)) == 0 || nw == -1) err(1, "stdout"); if (nr < 0) { warn("%s", filename); rval = 1; } }
No comments yet.